
- C Programming Tutorial
- Basics of C
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Type Casting
- C - Booleans
- Constants and Literals in C
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- Operators in C
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- Decision Making in C
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- Functions in C
- C - Functions
- C - Main Function
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- Scope Rules in C
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- Arrays in C
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- Pointers in C
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- Strings in C
- C - Strings
- C - Array of Strings
- C - Special Characters
- C Structures and Unions
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Lookup Tables
- C - Dot (.) Operator
- C - Enumeration (or enum)
- C - Structure Padding and Packing
- C - Nested Structures
- C - Anonymous Structure and Union
- C - Bit Fields
- C - Typedef
- File Handling in C
- C - Input & Output
- C - File I/O (File Handling)
- C Preprocessors
- C - Preprocessors
- C - Pragmas
- C - Preprocessor Operators
- C - Header Files
- Memory Management in C
- C - Memory Management
- C - Memory Address
- C - Storage Classes
- Miscellaneous Topics
- C - Error Handling
- C - Variable Arguments
- C - Command Execution
- C - Math Functions
- C - Static Keyword
- C - Random Number Generation
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Cheat Sheet
- C - Useful Resources
- C - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary

Pointer to Pointer (Double Pointer) in C
What is a double pointer in c.
A pointer to pointer which is also known as a double pointer in C is used to store the address of another pointer.
A variable in C that stores the address of another variable is known as a pointer . A pointer variable can store the address of any type including the primary data types, arrays, struct types, etc. Likewise, a pointer can store the address of another pointer too, in which case it is called "pointer to pointer" (also called "double pointer" ).
A "pointer to a pointer" is a form of multiple indirection or a chain of pointers. Normally, a pointer contains the address of a variable. When we define a "pointer to a pointer", the first pointer contains the address of the second pointer, which points to the location that contains the actual value as shown below −

Declaration of Pointer to a Pointer
The declaration of a pointer to pointer ( double pointer ) is similar to the declaration of a pointer, the only difference is that you need to use an additional asterisk ( * ) before the pointer variable name.
For example, the following declaration declares a "pointer to a pointer" of type int −
When a target value is indirectly pointed to by a "pointer to a pointer", accessing that value requires that the asterisk operator be applied twice.
Example of Pointer to Pointer (Double Pointer)
The following example demonstrates the declaration, initialization, and using pointer to pointer (double pointer) in C:
How Does a Normal Pointer Work in C?
Assume that an integer variable "a" is located at an arbitrary address 1000. Its pointer variable is "b" and the compiler allocates it the address 2000. The following image presents a visual depiction −

Let us declare a pointer to int type and store the address of an int variable in it.
The dereference operator fetches the value via the pointer.
Here is the complete program that shows how a normal pointer works −
It will print the value of int variable, its address, and the value obtained by the dereference pointer −
How Does a Double Pointer Work?
Let us now declare a pointer that can store the address of "b", which itself is a pointer to int type written as "int *".
Let's assume that the compiler also allocates it the address 3000.

Hence, "c" is a pointer to a pointer to int, and should be declared as "int **".
You get the value of "b" (which is the address of "a"), the value of "c" (which is the address of "b:), and the dereferenced value from "c" (which is the address of "a") −
Here, "c" is a double pointer. The first asterisk in its declaration points to "b" and the second asterisk in turn points to "a". So, we can use the double reference pointer to obtain the value of "a" from "c".
This should display the value of 'a' as 10.
Here is the complete program that shows how a double pointer works −
Run the code and check its output −
A Double Pointer Behaves Just Like a Normal Pointer
A "pointer to pointer" or a "double pointer" in C behaves just like a normal pointer. So, the size of a double pointer variable is always equal to a normal pointer.
We can check it by applying the sizeof operator to the pointers "b" and "c" in the above program −
This shows the equal size of both the pointers −
Note: The size and address of different pointer variables shown in the above examples may vary, as it depends on factors such as CPU architecture and the operating system. However, they will show consistent results.
Multilevel Pointers in C (Is a Triple Pointer Possible?)
Theoretically, there is no limit to how many asterisks can appear in a pointer declaration.
If you do need to have a pointer to "c" (in the above example), it will be a "pointer to a pointer to a pointer" and may be declared as −
Mostly, double pointers are used to refer to a two−dimensional array or an array of strings.
- C Programming Tutorial | What is C Language
- History of C
- Features of C
- How to install C
- First C Program
- Compilation Process in C
- printf scanf
- Variables in C
- Data Types in c
- Keywords in c
- C Identifiers
- C Operators
- C Format Specifier
- C Escape Sequence
- ASCII value in C
- Constants in C
- Literals in C
- Tokens in C
- Static in C
- Programming Errors in C
- Compile time vs Runtime
- Conditional Operator in C
- Bitwise Operator in C
- 2s complement in C
C Fundamental Test
C control statements.
- if-else vs switch
- C do-while loop
- C while loop
- Nested Loops in C
- Infinite Loop in C
- Type Casting
- C Control Statement Test
C Functions
- What is function
- Call: Value & Reference
- Recursion in c
- Storage Classes
- C Functions Test
- Return an Array in C
- Array to Function
C Array Test
- C Pointer to Pointer
- C Pointer Arithmetic
- Dangling Pointers in C
- sizeof() operator in C
- const Pointer in C
- void pointer in C
- C Dereference Pointer
- Null Pointer in C
- C Function Pointer
- Function pointer as argument in C
C Pointers Test
C dynamic memory.
- Dynamic memory
- String in C
- C gets() & puts()
- C String Functions
C String Test
- C Math Functions
C Structure Union
- C Structure
- typedef in C
- C Array of Structures
- C Nested Structure
- Structure Padding in C
C Structure Test
- C File Handling
- C fprintf() fscanf()
- C fputc() fgetc()
- C fputs() fgets()
- C Preprocessor
C Interview
- C Interview Questions
- C Fundamental 1
- C Fundamental 2
- C Fundamental 3
- C Fundamental 4
C Control Test
- C Control Statement 1
- C Control Statement 2
- C Control Statement 3
- C Control Statement 4
C Function Test
- C Functions 1
- C Functions 2
- C Functions 3
- C Functions 4
- C Pointers 1
- C Pointers 2
- C Pointers 3
- C Pointers 4
- C Structure 1
- C Structure 2
- C Structure 3
- C Structure 4
- C Preprocessor 1
- C Preprocessor 2
- C Preprocessor 3
- C Preprocessor 4
Latest Courses
We provides tutorials and interview questions of all technology like java tutorial, android, java frameworks
Contact info
G-13, 2nd Floor, Sec-3, Noida, UP, 201301, India
[email protected] .
Latest Post
PRIVACY POLICY
Interview Questions
Online compiler.
- Engineering Mathematics
- Discrete Mathematics
- Operating System
- Computer Networks
- Digital Logic and Design
- C Programming
- Data Structures
- Theory of Computation
- Compiler Design
- Computer Org and Architecture
Pointer Expressions in C with Examples
Prerequisite: Pointers in C Pointers are used to point to address the location of a variable. A pointer is declared by preceding the name of the pointer by an asterisk(*) . Syntax:
When we need to initialize a pointer with variable’s location, we use ampersand sign(&) before the variable name. Example:
The ampersand (&) is used to get the address of a variable. We can directly find the location of any identifier by just preceding it with an ampersand(&) sign. Example:
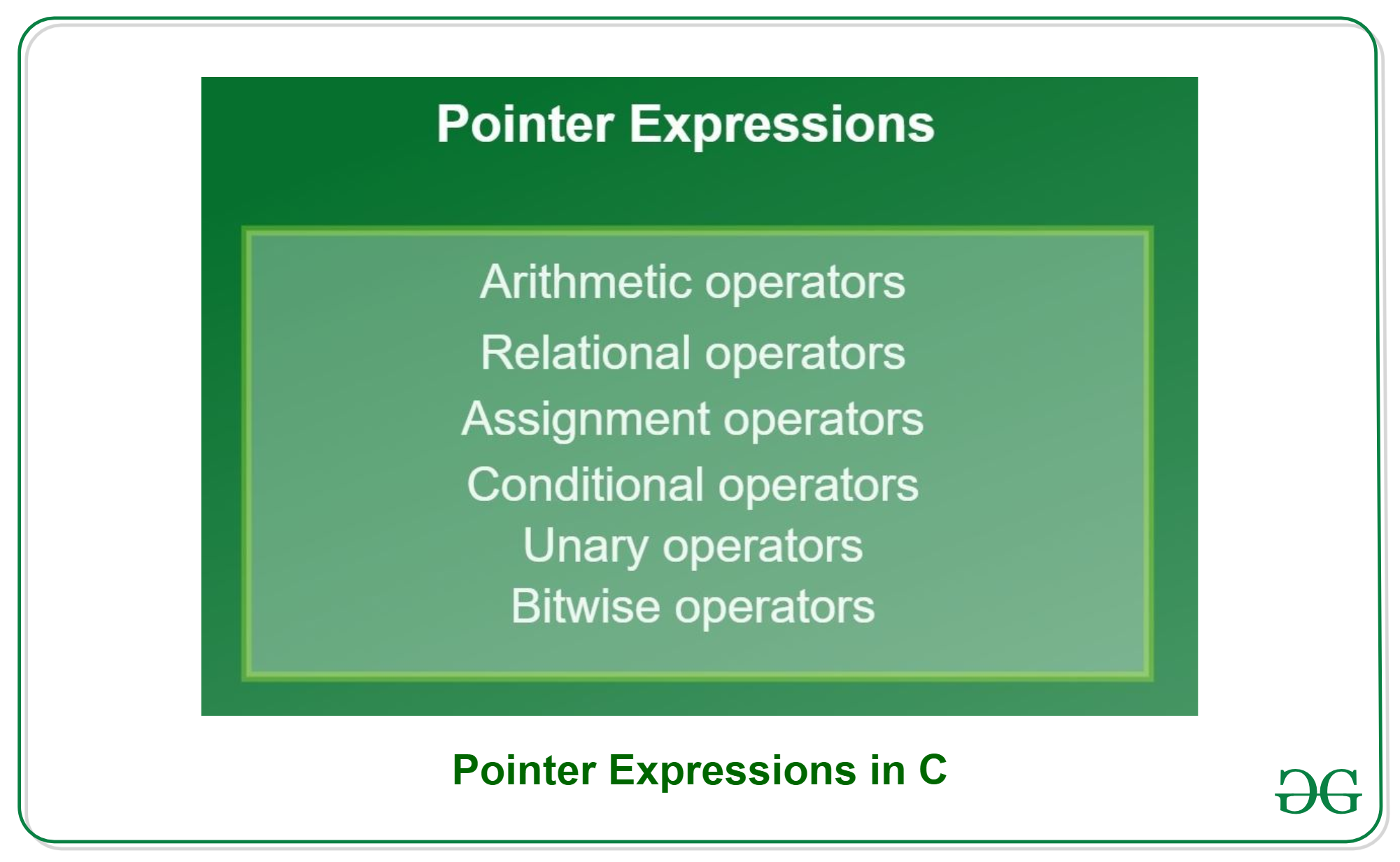
Arithmetic Operators
We can perform arithmetic operations to pointer variables using arithmetic operators. We can add an integer or subtract an integer using a pointer pointing to that integer variable. The given table shows the arithmetic operators that can be performed on pointer variables:
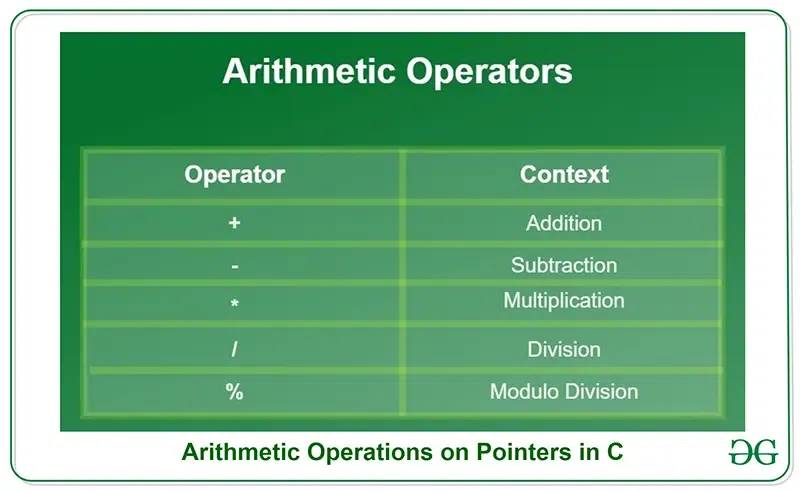
We can also directly perform arithmetic expressions on integers by dereferencing pointers. Let’s look at the example given below where p1 and p2 are pointers.
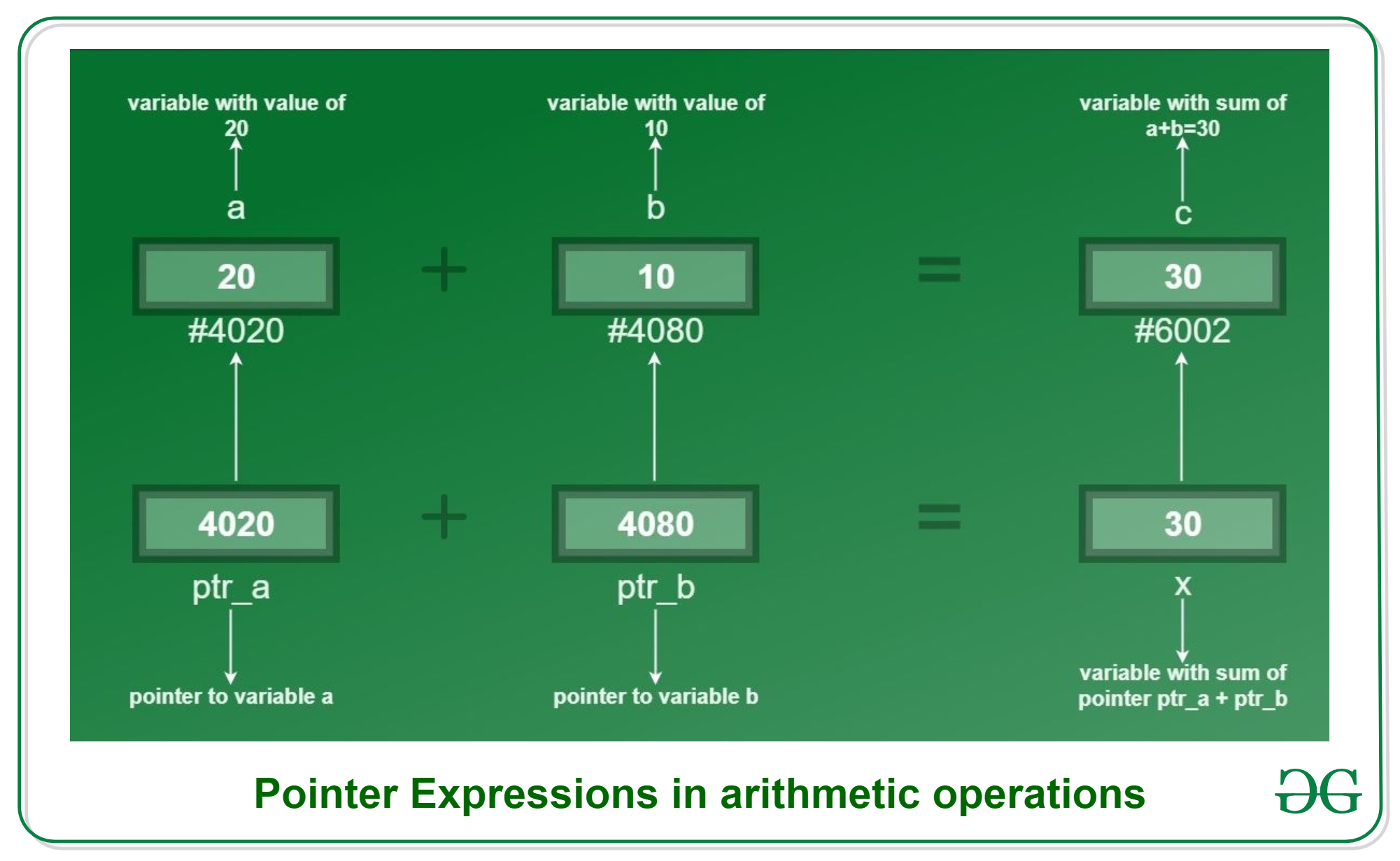
Note: While performing division, make sure you put a blank space between ‘/’ and ‘*’ of the pointer as together it would make a multi-line comment(‘/*’). Example:
Relational Operators
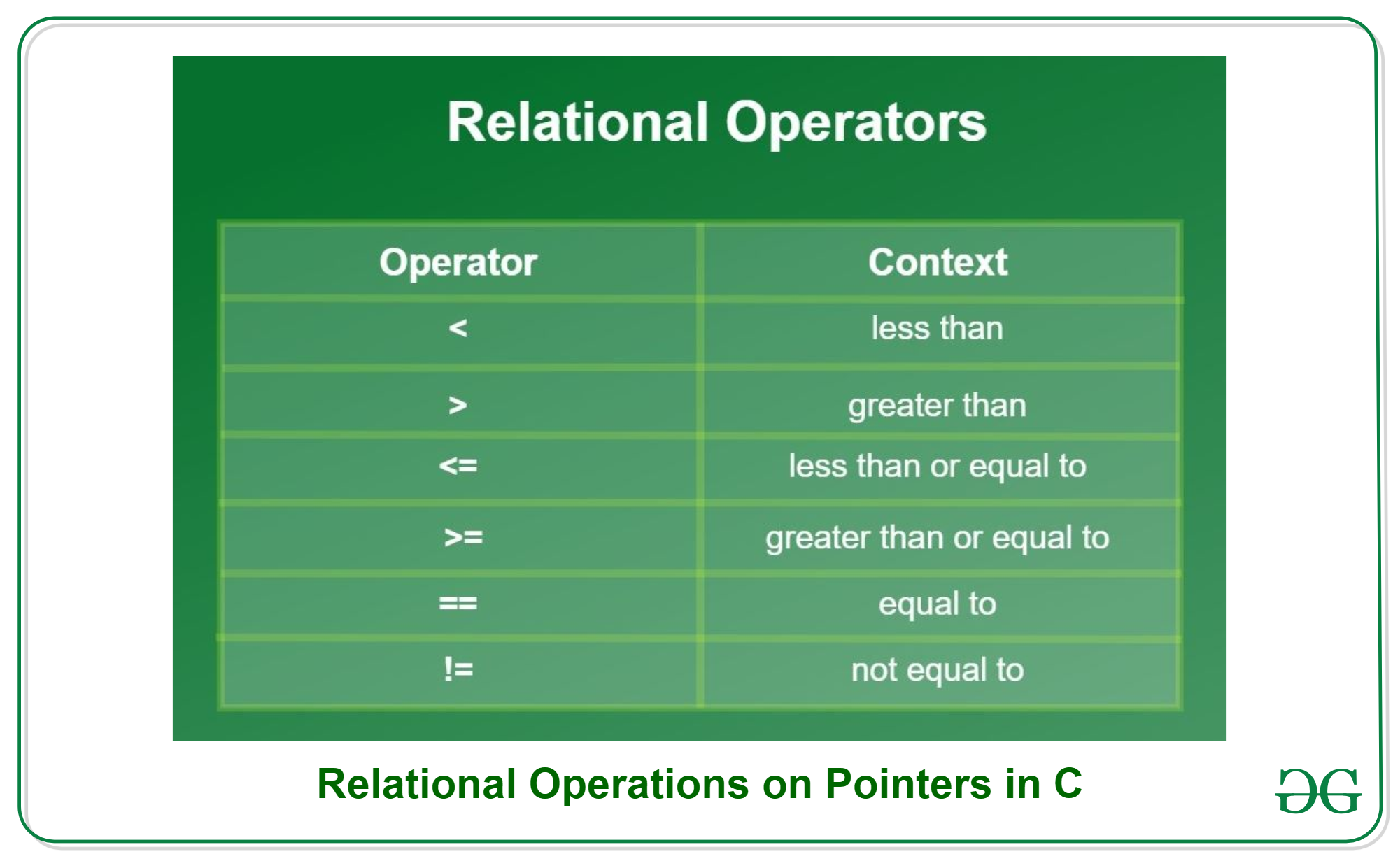
The value of the relational expression is either 0 or 1 that is false or true. The expression will return value 1 if the expression is true and it’ll return value 0 if false. Let us understand relational expression on pointer better with the code given below:
Assignment Operators
Assignment operators are used to assign values to the identifiers. There are multiple shorthand operations available. A table is given below showing the actual assignment statement with its shorthand statement.
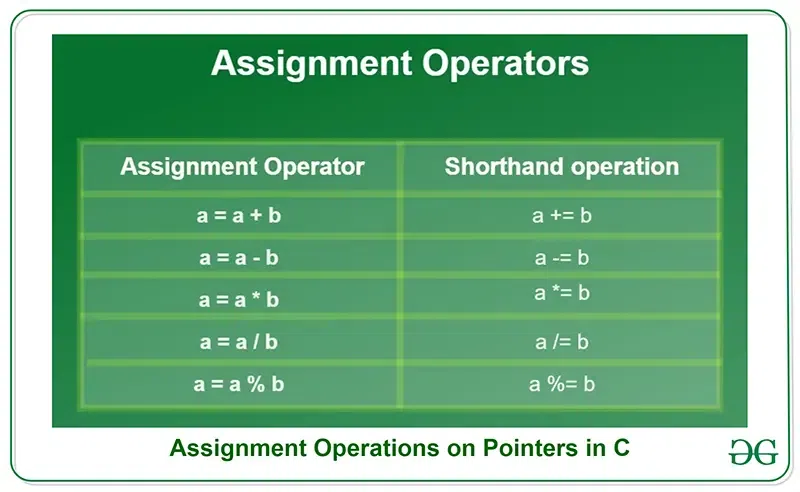
Let us understand assignment operator in better way with the help of code given below:
Conditional Operators
There is only one mostly used conditional operator in C known as Ternary operator. Ternary operator first checks the expression and depending on its return value returns true or false, which triggers/selects another expression. Syntax:
- As shown in example, assuming *ptr1=20 and *ptr2=10 then the condition here becomes true for the expression, so it’ll return value of true expression i.e. *ptr1, so variable ‘c’ will now contain value of 20.
- Considering same example, assume *ptr1=30 and *ptr2=50 then the condition is false for the expression, so it’ll return value of false expression i.e. *ptr2, so variable ‘c’ will now contain value 50.
Let us understand the concept through the given code:
Unary Operators
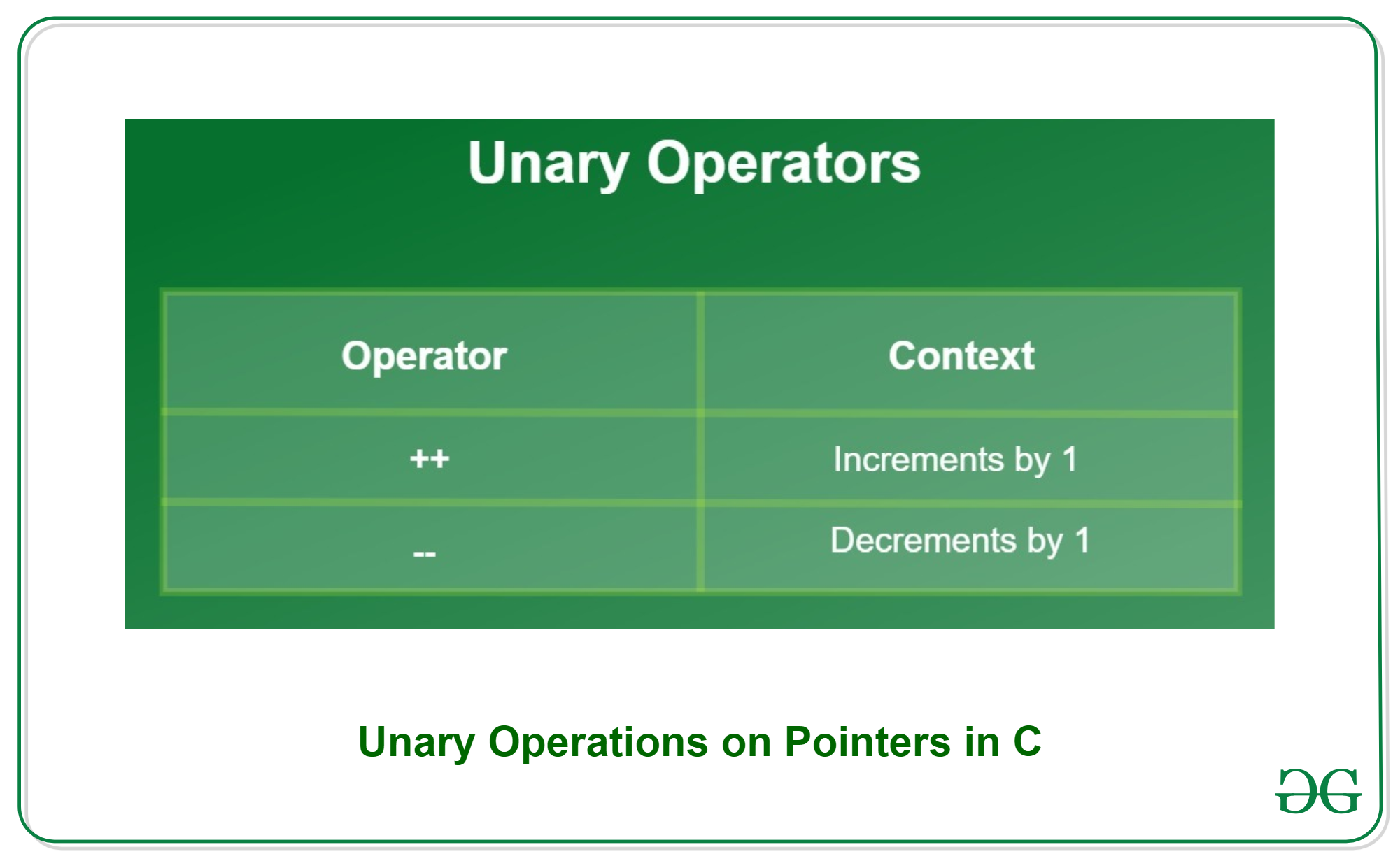
Let us understand the use of the unary operator through the given code:
Bitwise Operators
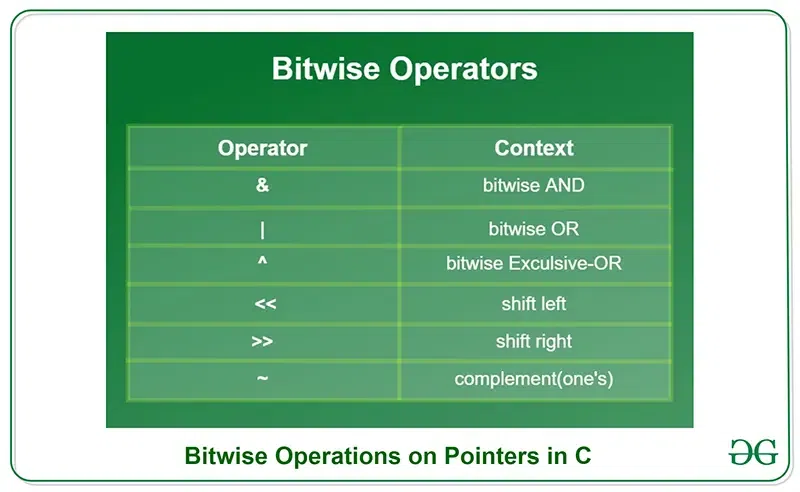
Similar Reads
Improve your coding skills with practice.
What kind of Experience do you want to share?
C – Pointer to Pointer (Double Pointer)
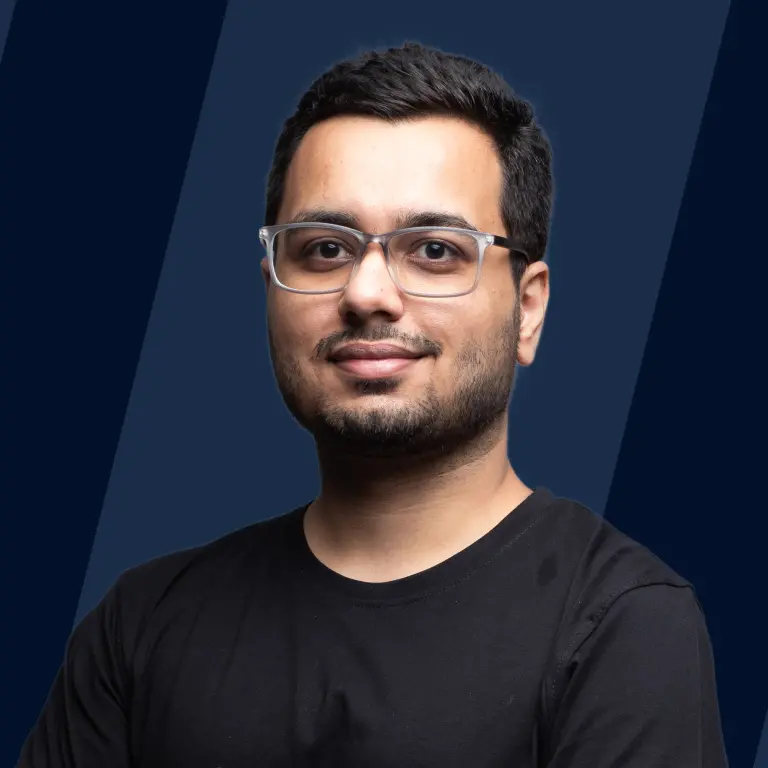
Similar to how a pointer variable in C can be used to access or modify the value of a variable in C, a pointer to pointer in C is used to access/modify the value of a pointer variable. Here, the "value" of the former pointer is as usual a memory address. So, using a pointer to the pointer aka a double pointer in C, we can make the previous pointer point to another memory location.
Prerequisite
- Pointers in C
Double Pointer Illustration
- In essence, a double pointer in C holds the memory address of another pointer. This means that it provides an indirect reference to the variable the first pointer points to. Imagine it as a chain where the double pointer points to another pointer, which in turn points to the actual variable.
Declaration of Pointer to Pointer in C
- To declare a double pointer, you utilize two asterisks before the variable name. Syntax: pointer_data_type **variable_name; For example:
- Trying to make a double pointer directly point to a normal variable, like with int **double_ptr = &var; , will lead to a compiler warning. The correct approach is to point to a regular pointer.

Example of Double Pointer in C
A double pointer, also known as a pointer to a pointer, is a powerful concept in C programming. It allows you to indirectly access and modify variables through multiple levels of indirection. Here's a basic example to illustrate the usage of double pointers:
In this example:
- We declare an integer variable value and initialize it with the value 42 .
- We declare a pointer ptr1 and make it point to the address of value .
- We declare a double pointer ptr2 and make it point to the address of ptr1 .
We then demonstrate how to access and modify the value of value using all three levels of indirection: directly using value , through ptr1 , and through ptr2 . Finally, we update the value through ptr2 and verify the change.
This example showcases the concept of double pointers and their ability to indirectly access and modify variables, making them a valuable tool in C programming.
How Double Pointer Works?
A double pointer, often referred to as a pointer to a pointer, is essentially a variable that stores the address of another pointer. In the C programming language, pointers are variables that hold memory addresses, typically of other variables. A double pointer takes this concept one level deeper.
Illustration
Imagine you have a box ( var ) that contains a value. Now, there's another box ( ptr ) that doesn't hold a direct value but rather a paper that tells you where the first box is located. This second box is a pointer. Now, imagine a third box ( double_ptr ), which instead of having a paper pointing to a direct value, has a paper pointing to the second box ( ptr ). This is the concept of a double pointer.
Explanation
Let's break it down step-by-step with code and explanation:
Regular Variable Declaration :
Here, an integer variable var is declared and assigned the value 10 .
Pointer Declaration :
An integer pointer ptr is declared, and it is assigned the address of the var variable.
Double Pointer Declaration :
Here, a double pointer double_ptr is declared, and it's assigned the address of the ptr pointer.
With this setup:
- *ptr will give the value 10 (value of var ).
- *double_ptr will give the address of var (because it points to ptr which holds the address of var ).
- **double_ptr will give the value 10 (value of var ).
In essence, a double pointer provides an indirect way to access the value of the original variable. This level of indirection becomes useful, especially when dealing with dynamic data structures like linked lists, trees, and 2D arrays.
Size of Pointer to Pointer in C
- Size is a key aspect to understand. While the data a pointer or double pointer refers to can be of various sizes, the pointers themselves typically have a fixed size, determined by the system architecture.
- On a 32-bit system, a pointer generally occupies 4 bytes, while on a 64-bit system, it's typically 8 bytes. This size remains consistent regardless of whether it's a single or double pointer.
Example 1: Program to find the size of a pointer to a pointer in C
Application of double pointers in c.
- Storing a list of strings : Double pointers can be used to create a dynamic array of strings, offering a space-efficient alternative to 2D arrays.
- Dynamic memory allocation : Especially useful when dealing with 2D arrays. Instead of fixed-size arrays, using double pointers allows for flexible dimensions.
- Command-line arguments : The main function in C accepts a double pointer, argv , which is an array of strings passed from the command line.
Multilevel Pointers in C
Multilevel pointers provide multiple layers of indirection in C. While double pointers are often used for applications like dynamic 2D arrays or arrays of strings, as we move beyond that, each additional level of indirection provides more flexibility but also introduces more complexity.
For instance:
- A single pointer ( *ptr ) points to a variable.
- A double pointer ( **ptr ) points to a single pointer, which in turn points to a variable.
- A triple pointer ( ***ptr ) points to a double pointer, which points to a single pointer, eventually leading to the variable.
And the pattern can continue, though, in practical applications, you'll rarely see pointers with more than two levels of indirection.
Syntax of Triple Pointer in C
The declaration of a triple pointer involves three asterisks before the variable name. Here's how you can declare and use a triple pointer:
In the above example, ***triple_ptr provides three levels of indirection to access the value of var . First, it accesses the memory location of double_ptr , then ptr , and finally var .
- Double pointers in C are very powerful and can have many applications (as explained in the Examples section) apart from simple data manipulation.
- In most cases, it is a personal preference whether to make use of a double pointer or use a workaround. However, in certain scenarios, the use of double pointers becomes mandatory. One such example is if we want to store a list of variable sized strings in a space efficient manner or if the size of a 2D array can change during the course of program execution.
- To change the value of a double pointer, we can use a "triple" pointer, which is a pointer to a pointer to a pointer (eg. int ***triple_ptr ). Similarly, to change the value of a triple pointer we can use a pointer to a pointer to a pointer to a pointer. In other words, to change the value of a "Level X" variable, we can use a "Level X+1" pointer. Thus, this concept can be extended to further levels.
- Utility Programs
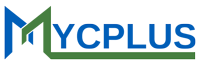
- C Tutorials
- C++ Tutorials
- C Code Examples
- C++ Code Examples
- C/C++ Programming Books
- Computer Science Books
- Software Programming Books
- Mobile Tech
Select Page
- A Complete Guide to using Double Pointer in C: Pointer-to-Pointer
Posted by M. Saqib | Updated Feb 11, 2024 | C Programming: Different Articles on C Programming |
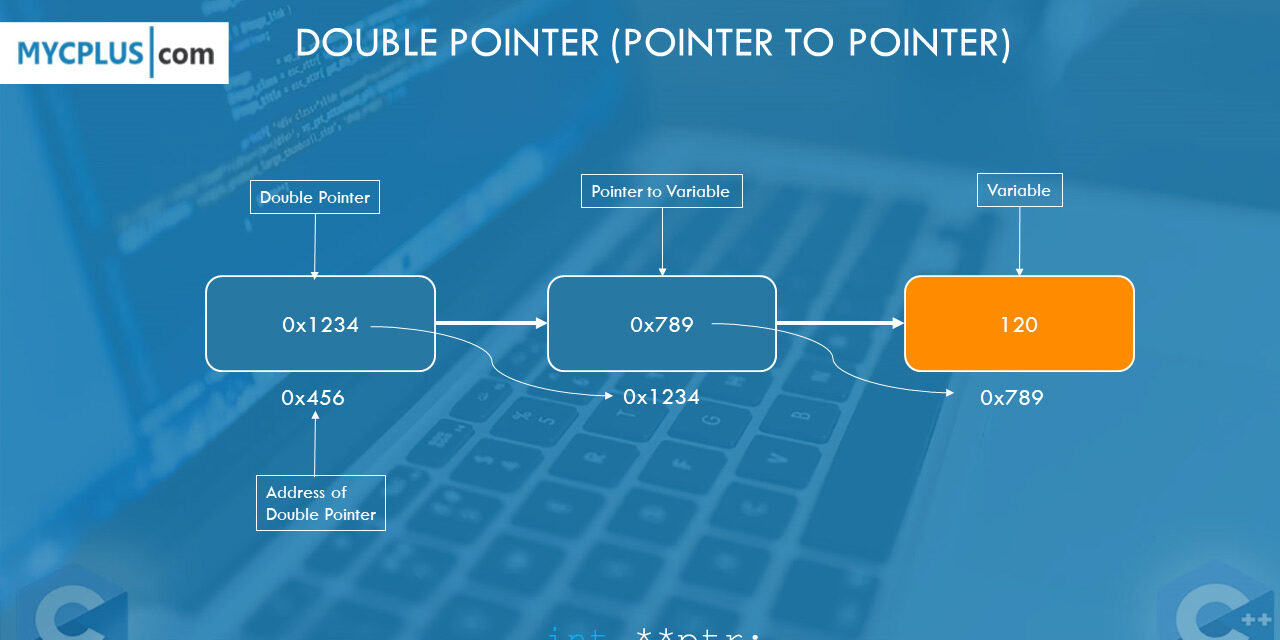
In C programming, a double pointer is a pointer that points to another pointer. It is also referred to as a pointer-to-pointer . A pointer in C is a variable that represents the location of an item, such as a variable or an array. We use pointers to pass information back and forth between a function and its reference point.
Sometimes, a pointer can be declared to point to another pointer which points to a variable. Here, the first pointer contains the address of the second pointer. The second pointer points to an actual memory location where the data is stored, i.e. a variable. That’s the reason why we also call such pointers as double pointers.
Table of Contents
How to declare a pointer-to-pointer, how to initialize a double pointer, c program to show how double pointer works, use double pointer to create two dimensional array, uses of pointer-to-pointer (double pointer), c programming language, 2nd edition, 21st century c: c tips from the new school, c programming absolute beginner’s guide, c programming: a modern approach, 2nd edition, programming arduino: getting started with sketches.
The syntax to declare a double pointer in C is:
In this example, the variable doubleptr is a double pointer to an integer value. The ** notation is used to indicate that doubleptr is a pointer to a pointer. To initialize a double pointer , you can use the address of operator & on a pointer variable, like this:
The following C program shows how pointer-to-pointer works by printing the pointer address as well as variable address and values:
The output of the above C Program is:
Value of var = 120 Value of var pointer = 120 Value of double pointer = 120 Address of var = 0x7ffe25192bfc Address of var pointer = 0x7ffe25192c00 Value in var pointer = 0x7ffe25192bfc Address of double pointer = 0x7ffe25192bfc Value in double pointer = 0x7ffe25192c00
Here is an example that demonstrates how to use double pointers to create a dynamic two-dimensional array. This program demonstrates how double pointers can be used to create complex data structures with dynamic memory allocation in C programming.
This program prompts the user to enter the number of rows and columns for a matrix (two dimensional array), then dynamically allocates memory for the matrix using double pointers. It fills the matrix with values, prints it to the console, and then frees the memory.
Double pointers serve a variety of purposes in C Programming. Here are some of the most common uses of double pointers and why you might want to use them:
- Dynamic Memory Allocation: Double pointers are often used to allocate memory for arrays, particularly multi-dimensional arrays. By using a double pointer, you can ensure that the memory allocation is protected even outside of a function call. To allocate space for a matrix or multi-dimensional arrays dynamically, you will need a double-pointer.
- Passing Handles Between Functions: This is particularly useful if you need to pass re-locatable memory between functions.
- Characters List: Another use is that if you want to have a list of characters (a word), you can use char *word, for a list of words (a sentence), you can use char **sentence, and for list of sentences (a paragraph), you can use char ***paragraph.
- Main Function Parameters: One of the most common uses (every C programmer should have encountered) as parameters to the main() function , i.e. int main(int argc, char **argv). Here argv is the array of arguments passed on to this program.
- Linked Lists: Double pointers are frequently used in linked list operations, such as insertion and deletion.
If you’re interested in learning more about double pointers, there are many helpful resources available online. You might start by reading some of the articles on StackOverflow or Quora .
Common Errors with Double Pointers in C
Here are some common errors with double pointers that new programmers make while write C Programming code:
- Incorrect Syntax: One common mistake when working with double pointers is using the wrong syntax. This can result in compilation errors or unexpected behavior in the program. For example, forgetting to include the ‘*’ operator when dereferencing a double pointer can cause the program to crash.
- Dereferencing Errors: Another common error is dereferencing incorrectly. This can happen when trying to access memory that hasn’t been allocated or has already been freed. Forgetting to check for NULL values can also lead to segmentation faults.
- Memory Allocation Errors: Double pointers are often used for dynamic memory allocation in C programming , but errors can occur when allocating memory. Common mistakes include not allocating enough memory, allocating too much memory, or failing to free memory when it’s no longer needed.
- Type Mismatch Errors: Double pointers can also cause type mismatch errors if they’re not properly cast to the correct type. This can happen when passing double pointers to functions or when assigning values to double pointers.
- Pointer Arithmetic Errors: Pointer arithmetic can be tricky in C programming, especially when working with double pointers. Common errors include using incorrect operators or not correctly accounting for the size of the data type.
By avoiding these common errors with double pointers in C programming, you can write better C programs. This will also help to improve your overall understanding of double pointers and enhance your programming skills further.
Here’s Must-Read List of C programming books for beginners
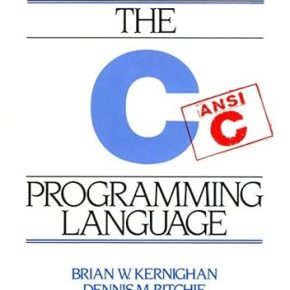
With over 600 5-star reviews on Amazon, readers agree that C Programming Language, 2nd Edition by Brian Kernighan and Dennis Ritchie is the best C Programming book for Beginners. The authors present the complete guide to ANSI standard C language programming. Written by the developers of C, this new version helps readers keep up with the finalized ANSI standard for C while showing how to take advantage of C’s rich set of operators, economy of expression, improved control flow, and data structures.
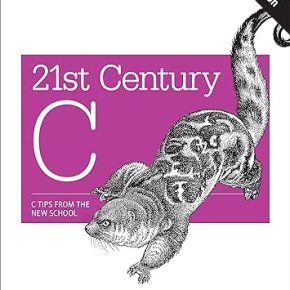
Throw out your old ideas about C and get to know a programming language that’s substantially outgrown its origins. With this revised edition of 21st Century C, you’ll discover up-to-date techniques missing from other C tutorials, whether you’re new to the language or just getting reacquainted.
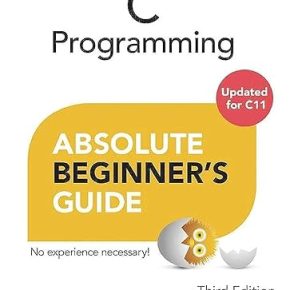
Greg Perry is the author of over 75 computer books and known for bringing programming topics down to the beginner’s level. His book C Programming Absolute Beginner’s Guide , is today’s best beginner’s guide to writing C programs–and to learning skills to use with practically any language. Its simple, practical instructions will help you start creating useful, reliable C code, from games to mobile apps. Plus, it’s fully updated for the new C11 standard and today’s free, open source tools!
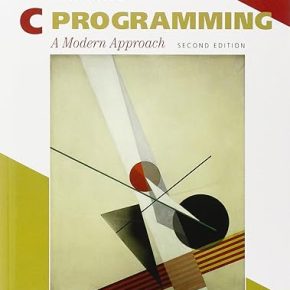
KN King tackles on some C standard library specifics header by header in his C Programming: A Modern Approach book. The second edition maintains all the book’s popular features and brings it up to date with coverage of the C99 standard. The new edition also adds a significant number of exercises and longer programming projects, and includes extensive revisions and updates.
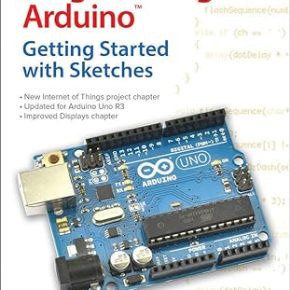
Simon Monk, a Ph.D. in software engineering, writes this great little book for learning to program the arduino using C language. This bestselling guide explains how to write well-crafted sketches using Arduino’s modified C language. You will learn how to configure hardware and software, develop your own sketches, work with built-in and custom Arduino libraries, and explore the Internet of Things—all with no prior programming experience required!
About The Author
Saqib is Master-level Senior Software Engineer with over 14 years of experience in designing and developing large-scale software and web applications. He has more than eight years experience of leading software development teams. Saqib provides consultancy to develop software systems and web services for Fortune 500 companies. He has hands-on experience in C/C++ Java, JavaScript, PHP and .NET Technologies. Saqib owns and write contents on mycplus.com since 2004.
Related Posts
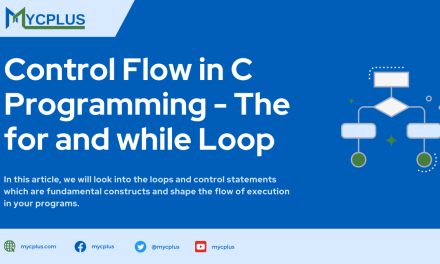
- Control Flow in C Programming – The for and while Loop
Updated Feb 12, 2024
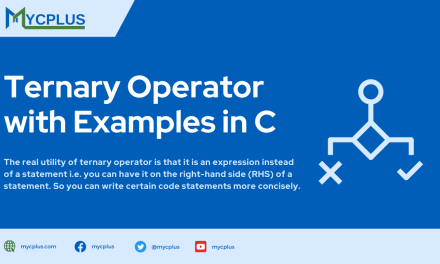
- Ternary Operator with examples in C
Updated Feb 11, 2024
![pointer to pointer assignment in c Difference between char[] and char* in C? Character Array and Pointer](https://cdn.mycplus.com/mycplus/wp-content/uploads/2020/09/char-array-and-char-pointer-in-C-440x264.png)
- Difference between char[] and char* in C? Character Array and Pointer
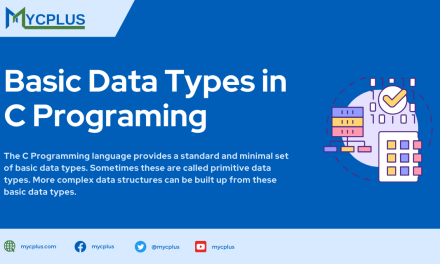
- Basic Data Types in C Programming
Updated Feb 21, 2024
- How to Master XML Conversion With C++
- C++23: Exploring the New Features
- Operators in C Programming
- Structure in C Programming
- Pure Virtual Functions in C++
- Understanding the Basics of C++ Programming
- What are the Best C++ Compilers to use in 2024?
- What are #ifndef and #define Directives?
- C++ Standard Template Library – List
- C++ Vector – std::vector – A Complete Guide with Examples and Programs
- File Handling in C++
- Ternary Operator with examples in C++
- Polymorphism in C++
- The C++ Modulus Operator [mod or % operator]
- Introduction to C++ – Lecture Notes
- An Introduction to C++
- Concurrency in C++ – A Course offered by University of Waterloo
- 256-Color VGA Programming in C
- Union in C Programming
- Advanced C++ Inheritance Techniques for Effective Object-Oriented Programming
- Inheritance in C++
- C++ Memory Management
- Transitioning from C to C++: A Quick Guide for Novice Programmers
- Understanding the Basics of Encapsulation in C++
- Functions in C++ Programming
- Learn File Handling Concepts in C Programming
- Virtual Functions in C++
- Multiple Inheritance in C++
- An overview of Generic Containers in C++
- Compounded Types in C++
- Pointers in C++
- Advanced Concepts and Patterns in Encapsulation
- Understanding C++ Templates: A Simplified Guide
- Graphics in C Language
- Working with Pointers in C
- Exception Handling in C++
- Operator Overloading in C++
- A Guide to Advanced Exception Handling in C++ Programming
- The Standard C++ Library
- Destructors in C++
- Constructors in C++
- Introduction to Classes in C++
- C++ and Object Orientation
- Generic Algorithms – Unlocking the Power of C++ STL Algorithms
- Strings in C++: A Complete Guide for Novice Programmers
Learn C practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, certification courses.
Created with over a decade of experience and thousands of feedback.
C Introduction
- Getting Started with C
- Your First C Program
C Fundamentals
- C Variables, Constants and Literals
- C Data Types
- C Input Output (I/O)
- C Programming Operators
C Flow Control
- C if...else Statement
- C while and do...while Loop
- C break and continue
- C switch Statement
- C goto Statement
- C Functions
- C User-defined functions
- Types of User-defined Functions in C Programming
- C Recursion
- C Storage Class
C Programming Arrays
- C Multidimensional Arrays
- Pass arrays to a function in C
C Programming Pointers
Relationship Between Arrays and Pointers
C Pass Addresses and Pointers
C Dynamic Memory Allocation
- C Array and Pointer Examples
- C Programming Strings
- String Manipulations In C Programming Using Library Functions
- String Examples in C Programming
C Structure and Union
C structs and Pointers
- C Structure and Function
C Programming Files
- C File Handling
- C Files Examples
C Additional Topics
- C Keywords and Identifiers
- C Precedence And Associativity Of Operators
- C Bitwise Operators
- C Preprocessor and Macros
- C Standard Library Functions
C Tutorials
- Access Array Elements Using Pointer
Pointers are powerful features of C and C++ programming. Before we learn pointers, let's learn about addresses in C programming.
- Address in C
If you have a variable var in your program, &var will give you its address in the memory.
We have used address numerous times while using the scanf() function.
Here, the value entered by the user is stored in the address of var variable. Let's take a working example.
Note: You will probably get a different address when you run the above code.
Pointers (pointer variables) are special variables that are used to store addresses rather than values.
Pointer Syntax
Here is how we can declare pointers.
Here, we have declared a pointer p of int type.
You can also declare pointers in these ways.
Let's take another example of declaring pointers.
Here, we have declared a pointer p1 and a normal variable p2 .
- Assigning addresses to Pointers
Let's take an example.
Here, 5 is assigned to the c variable. And, the address of c is assigned to the pc pointer.
Get Value of Thing Pointed by Pointers
To get the value of the thing pointed by the pointers, we use the * operator. For example:
Here, the address of c is assigned to the pc pointer. To get the value stored in that address, we used *pc .
Note: In the above example, pc is a pointer, not *pc . You cannot and should not do something like *pc = &c ;
By the way, * is called the dereference operator (when working with pointers). It operates on a pointer and gives the value stored in that pointer.
- Changing Value Pointed by Pointers
We have assigned the address of c to the pc pointer.
Then, we changed the value of c to 1. Since pc and the address of c is the same, *pc gives us 1.
Let's take another example.
Then, we changed *pc to 1 using *pc = 1; . Since pc and the address of c is the same, c will be equal to 1.
Let's take one more example.
Initially, the address of c is assigned to the pc pointer using pc = &c; . Since c is 5, *pc gives us 5.
Then, the address of d is assigned to the pc pointer using pc = &d; . Since d is -15, *pc gives us -15.
- Example: Working of Pointers
Let's take a working example.
Explanation of the program
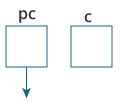
Common mistakes when working with pointers
Suppose, you want pointer pc to point to the address of c . Then,
Here's an example of pointer syntax beginners often find confusing.
Why didn't we get an error when using int *p = &c; ?
It's because
is equivalent to
In both cases, we are creating a pointer p (not *p ) and assigning &c to it.
To avoid this confusion, we can use the statement like this:
Now you know what pointers are, you will learn how pointers are related to arrays in the next tutorial.
Table of Contents
- What is a pointer?
- Common Mistakes
Sorry about that.
Our premium learning platform, created with over a decade of experience and thousands of feedbacks .
Learn and improve your coding skills like never before.
- Interactive Courses
- Certificates
- 2000+ Challenges
Related Tutorials

COMMENTS
We can use a pointer to a pointer to change the values of normal pointers or create a variable-sized 2-D array. A double pointer occupies the same amount of space in the memory stack as a normal pointer.
int variable; int *ptr = &variable; *ptr = 20; printf("%d", *ptr); return 0; } Here, the int variable isn't initialized—but that's fine, because you're just going to replace whatever value was there with 20. The key is that the pointer is initialized to point to the variable.
A pointer variable can store the address of any type including the primary data types, arrays, struct types, etc. Likewise, a pointer can store the address of another pointer too, in which case it is called "pointer to pointer" (also called "double pointer").
C Double Pointer (Pointer to Pointer) As we know that, a pointer is used to store the address of a variable in C. Pointer reduces the access time of a variable. However, In C, we can also define a pointer to store the address of another pointer.
Pointers in C are variables that are used to store the memory address of another variable. Pointers allow us to efficiently manage the memory and hence optimize our program. In this article, we will discuss some of the major applications of pointers in C. Prerequisite: Pointers in C. C Pointers ApplicationThe following are some major applications o
Syntax: datatype *pointer_name; When we need to initialize a pointer with variable’s location, we use ampersand sign (&) before the variable name. Example: C. int var=10; int *pointer=&var; The ampersand (&) is used to get the address of a variable.
A double pointer, also known as a pointer to a pointer, is a powerful concept in C programming. It allows you to indirectly access and modify variables through multiple levels of indirection. Here's a basic example to illustrate the usage of double pointers:
How to declare a pointer-to-pointer? The syntax to declare a double pointer in C is: 1. int **doubleptr; In this example, the variable doubleptr is a double pointer to an integer value. The ** notation is used to indicate that doubleptr is a pointer to a pointer.
A Simple Example of Pointers in C. This program shows how a pointer is declared and used. There are several other things that we can do with pointers, we have discussed them later in this guide. For now, we just need to know how to link a pointer to the address of a variable.
Assigning addresses to Pointers. Let's take an example. int* pc, c; c = 5; pc = &c; Here, 5 is assigned to the c variable. And, the address of c is assigned to the pc pointer. Get Value of Thing Pointed by Pointers. To get the value of the thing pointed by the pointers, we use the * operator.